Decision making is an important part of programming. This can be achieved in R programming using the conditional if...else statement.
R if statement
The syntax of if
statement is:
if (test_expression) {
statement
}
If test_expression
is TRUE
, the statement gets executed. But if it's FALSE
, nothing happens.
Here, test_expression
can be a logical or numeric vector, but only the first element is taken into consideration.
In the case of a numeric vector, zero is taken as FALSE
, rest as TRUE
.
Flowchart of if statement
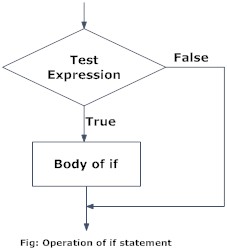
Example 1: if statement
x <- 5
if(x > 0){
print("Positive number")
}
Output
[1] "Positive number"
if else statement
The syntax of if else
statement is:
if (test_expression) {
statement1
} else {
statement2
}
The else
part is optional and is only evaluated if test_expression
is FALSE
.
Note: The else
must be in the same line as the closing braces of the if
statement.
Flowchart of if else statement
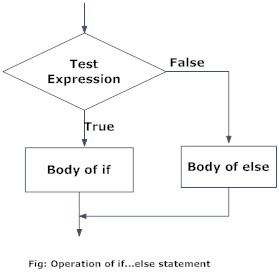
Example 2: if else statement
x <- -5
if(x > 0){
print("Non-negative number")
} else {
print("Negative number")
}
Output
[1] "Negative number"
The above conditional can also be written in a single line as follows:
if(x > 0) print("Non-negative number") else print("Negative number")
This feature of R allows us to write constructs as shown below:
x <- -5
y <- if(x > 0) 5 else 6
y
Output
[1] 6
Here, -5 is not greater than 0, so the condition of the if
statement evaluates to false. Hence, the code proceeds to the else
statement and assigns the value 6 to the variable y
.
if else Ladder
The if…else
ladder (if..else..if) statement allows you to execute a block of code among more than 2 alternatives.
The syntax of for this is:
if ( test_expression1) {
statement1
} else if ( test_expression2) {
statement2
} else if ( test_expression3) {
statement3
} else {
statement4
}
Only one statement will get executed depending upon the test expressions.
Example 3: nested if else
x <- 0
if (x < 0) {
print("Negative number")
} else if (x > 0) {
print("Positive number")
} else
print("Zero")
Output
[1] "Zero"
The variable x
is assigned the value 0, which is not less than 0, so the condition x < 0
evaluates to false.
Since the condition in the else if
statement is also false, the code proceeds to the else
statement and executes the corresponding block, resulting in the output "Zero"
.
There is an easier way to use the if else statement specifically for vectors in R programming.
You can use the ifelse()
function instead; the vector equivalent form of the if else statement.
Check out these related examples: