A loop executes a block of code for multiple times. But, sometimes this flow of the loop needs to be skipped or terminated. So, for alteration in the normal flow of the loop we use the break
and continue
statements.
break
- terminates the loopcontinue
- skips the current iteration of the loop and moves on to the next
Before you proceed with this tutorial, be sure to learn about the for loop and while loop first.
Python break Statement
The break
statement is used to terminate or end the loop completely. The control of the program flows to the statement immediately after the body of the loop.
Let's see an example of it:
n = 1;
while True:
print(n)
n = n + 1
break
print('This is outside the loop.')
Output
1 This is outside the loop.
Working of break Statement in Python
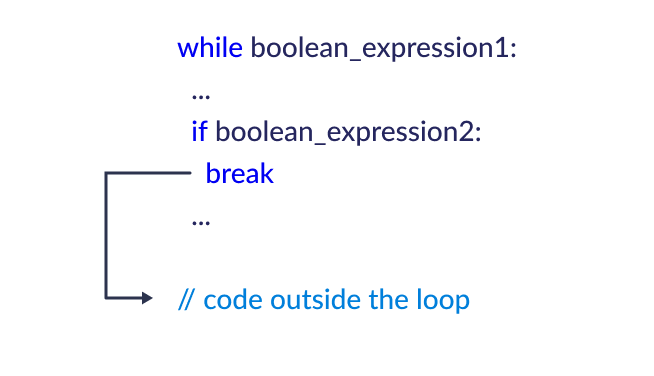
Note: The break
statement is usually used with decision making statements such as if...else.
Example : Python break Statement
# the loop terminates when item is equal to 5
for item in range(1, 7):
if item == 5:
break
print(item)
print('Outside of the loop')
Output
1 2 3 4 Outside of the loop
In this program, we iterate through the sequence of numbers in range 1 to 7. And in each iteration we check if the item == 5
, if the condition is true
then it breaks the loop otherwise the item
gets printed.
Here in the output, you can see the sequence of numbers from 1 to 4 is printed. However, the remaining sequence of numbers is not printed.
This is because the loop terminates when item == 5
, causing the control of the program to exit the loop. After the loop ends, the statement Outside of the loop
is printed.
Python continue Statement
The continue
statement in Python skips the rest of the code inside the loop for
the current iteration.
The loop will not terminate but continues on with the next iteration.
Working of continue Statement in Python
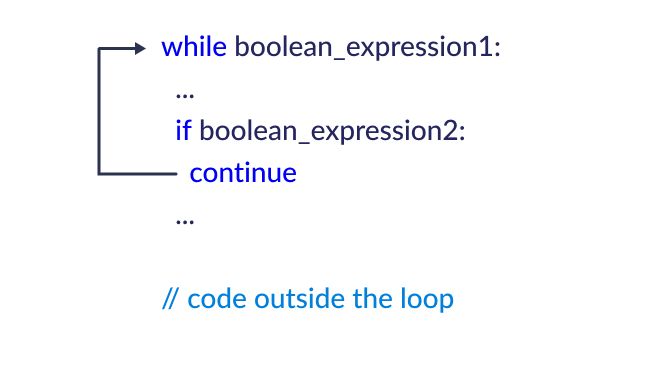
Example: Python continue Statement
for item in range(1, 7):
# skips the current iteration when item is equal to 5
if item == 5:
continue
print(item)
print('Outside of loop')
Output
1 2 3 4 6 Outside of loop
This program is the same as the above example except the break
statement has been replaced with continue
.
Here, you can see the sequence of numbers from 1 to 7 is printed but 5 is skipped because the continue
statement skips the rest of the code inside a loop and continues with the next iteration.
Hence 5 is skipped. But the number after that is printed and the statement Outside of the loop
is printed finally.
Recommended Reading: Python for loop