In Python, a set is a collection of unordered items. Every item of a set is unique (no duplicates) and immutable. However, the set itself is mutable; we can add or remove set items.
Creating Set
A set is created by placing items inside curly braces, {}
, separated by commas.
animals = {'dog', 'cat', 'tiger'}
print(animals) # {'tiger', 'dog', 'cat'}
Note: The order of items in output may be different than how we assigned them. It's because sets are unordered.
If we add duplicate items to a set, duplicates will be removed
animals = {'dog', 'cat', 'tiger', 'dog', 'tiger'}
print(animals) # {'tiger', 'cat', 'dog'}
Here, we can see 'dog'
and 'tiger'
only once in the output because sets don't allow duplicate values.
Creating an Empty Set
We cannot use {}
to create an empty set. It's because this will create an empty dictionary. To create an empty set, we use the set()
function.
# creating empty set
animals = set()
print(animals) # set()
Add Items to a Set
Sets in Python are mutable. We can add and remove items from them.
To add a single item to a set, we use the add()
method.
animals = {'dog', 'cat'}
# adding 'monkey' to the set
animals.add('monkey')
print(animals) # {'monkey', 'cat', 'dog'}
Add Items of Iterables (Lists, Tuples etc.)
We can use the update()
method to add items from other iterables to a set.
animals = {'dog', 'cat'}
wild_animals = {'tiger', 'lion'}
# adding items from the wild_animals iterable
animals.update(wild_animals)
print(animals) # {'dog', 'lion', 'cat', 'tiger'}
Remove Items from a Set
We can use either the discard()
method or the remove()
method to remove an item from a set.
The difference between discard()
and remove()
is that
discard()
returnsNone
if the item is not in a setremove()
throws an error if the item is not in a set
Using discard()
animals = {'dog', 'cat', 'tiger'}
# removing 'tiger'
animals.discard('tiger')
print(animals) # {'dog', 'cat'}
# trying to remove 'ferret'
# doesn't throw error
animals.discard('ferret')
print(animals) # {'cat', 'dog'}
Using remove()
animals = {'dog', 'cat', 'tiger'}
# removing 'tiger'
animals.remove('tiger')
print(animals) # {'dog', 'cat'}
# trying to remove 'ferret'
# throws an error
animals.remove('ferret') KeyError: 'ferret'
print(animals) # {'cat', 'dog'}
Remove all Items from a Set
We can use the clear()
method to remove all items from a set.
animals = {'dog', 'cat', 'tiger'}
# remove all items
animals.clear()
print(animals) # set()
Python Set Operations
Python sets have the same properties as that of sets in mathematics. Let's look at some set operations.
Union of Sets
The union of two sets is a set of all items in both sets. To find the union of sets, we can either use the union()
method or the pipe symbol, |
.
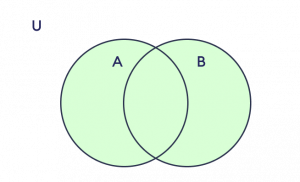
domestic_animals = {'dog', 'cat', 'elephant'}
wild_animals = {'lion', 'tiger', 'elephant'}
# finding the union of sets
animals = domestic_animals | wild_animals
print(animals)
# Output
# {'lion', 'elephant', 'cat', 'dog', 'tiger'}
Intersection of Sets
The intersection of two sets is a set of items that are common in both sets.
To find the intersection of sets, we can either use the intersection()
method or the ampersand symbol, &
.
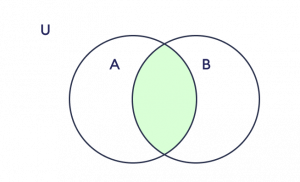
domestic_animals = {'dog', 'cat', 'elephant'}
wild_animals = {'lion', 'tiger', 'elephant'}
# finding the intersection of sets
common_animals = domestic_animals & wild_animals
print(common_animals) # {'elephant'}
Other Set Operations
We can find
- the difference of sets using either the
-
operator or thedifference()
method. - the symmetric difference of sets using either the
^
operator or thesymmetric_difference()
method.
Python Set Methods
Python has many useful methods that make it really easy to work with sets. Here are the commonly used set methods.
Method | Description |
---|---|
add() |
adds an item to the set |
clear() |
removes all items from the set |
copy() |
returns the shallow copy of the set |
difference() |
returns the difference of two sets |
discard() |
removes the specified item from the set |
intersection() |
returns the intersection of two or more sets |
pop() |
removes an arbitrary item from the set |
remove() |
removes the specified item from the set |
symmetric_difference() |
returns the symmetric difference of sets |
union() |
returns the union of two or more sets |
update() |
adds items from an iterable to the set |
Python Set Length
We can find the number of items in a set using the len()
function. For example,
animals = {'dog', 'cat', 'tiger'}
print(len(animals)) # 3
Iterating Through a Set
We can iterate through elements of a set using the for loop.
animals = {'dog', 'cat', 'tiger'}
for animal in animals:
print(animal)
Output
tiger cat dog
Note: Since sets are unordered, the order in which the items are printed is random.
Python Set Summary
In Python, a set has the following features:
- unordered - items are in random order
- unique - items of a set are unique
- mutable - can add or remove items
Recommended Reading: Python Dictionary