Python Packages
A package is a directory containing multiple modules and other sub-packages.
Suppose we are developing a game with multiple objects, so it may have these different modules.
player.py
boss.py
gun.py
knife.py
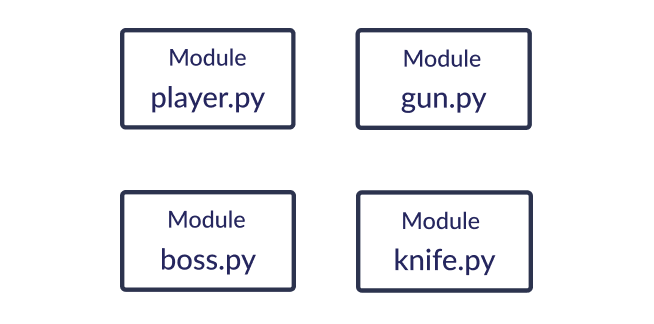
Since these modules are in the same location, they look cluttered. We can structure them in this way:
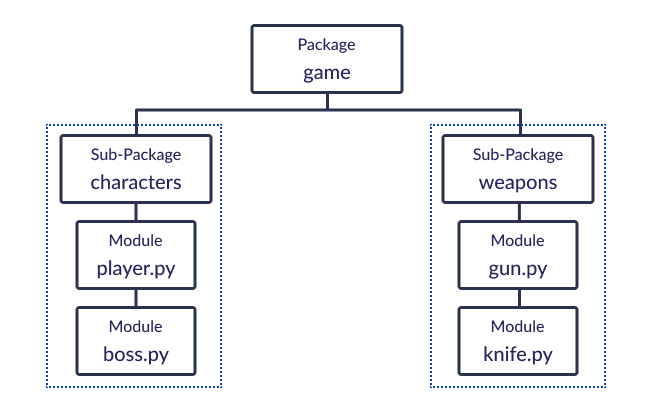
Here, the player
and boss
modules are similar (they both deal with game characters). So, they are kept under the characters
package. Similarly, the gun
and knife
modules are kept inside the weapons
package.
Then, both characters and weapons packages are kept inside the main package, i.e., game
package.
As you can see, our project looks much more organized and structured with the use of packages.
Let's implement this in code with the following steps:
- Create a directory named
game
that will contain all our game components. - We also need to create an
__init__.py
file inside game directory. This special file tells Python that this directory is a Python package. - Also create the
characters
package with an__init__.py
file. - Now, create
player.py
andboss.py
modules inside thecharacters
package.
In player.py
:
def get_player_info():
print("I am the main player.")
In boss.py
:
def get_boss_info():
print("I am the enemy player.")
While developing large programs, these modules might contain classes and multiple functions.
Let's create a main.py
file outside the game
directory.
You can now import and use them in the following ways:
import game.characters.player
game.characters.player.get_player_info()
Output
I am the main player.
from game.characters import player
player.get_player_info()
Output
I am the main player.
from game.characters.boss import get_boss_info
get_boss_info()
Output
I am the enemy player.
import game.characters.player
import game.characters.boss
game.characters.player.get_player_info()
game.characters.boss.get_boss_info()
Output
I am the main player. I am the enemy player.
from game.characters import player
from game.characters import boss
player.get_player_info()
boss.get_boss_info()
Output
I am the main player. I am the enemy player.
from game.characters.player import get_player_info
from game.characters.boss import get_boss_info
get_player_info()
get_boss_info()
Output
I am the main player. I am the enemy player.
Note: When you use packages, always try to give descriptive names to functions and classes so that you don't get confused.
Using __init__.py
The code inside __init__.py
runs automatically when we import the package.
Let's add a print statement in this file inside the game
package.
print("Initializing game features...")
Let's run this code in main.py
and see what output we get:
from game.characters import player
from game.characters.boss import get_boss_info
player.get_player_info()
get_boss_info()
Output
Initializing game features... I am the main player. I am the enemy player.
Python pip
There are thousands of useful packages tailored for specific tasks that are developed and maintained by the active Python community.
When you start working on more advanced projects, you will have to use these packages at some point rather than building everything from scratch.
For example, if you are working with web development, you will probably use packages like django
or flask
.
pip
is the standard package manager for Python. It helps to install and manage additional packages that are not available in the Python standard library.
Installing packages
Use the following command in your terminal or command prompt to install Python packages:
pip install <package_name>
For example, the command below installs the pandas
package, a popular package for data analysis in Python.
pip install pandas
Installing Specific version of a package
To install version 2.21.0 of requests
package,
pip install requests==2.21.0
List all packages with pip
pip list
Remove Packages with pip
pip uninstall numpy
This uninstalls the numpy
package from our machine.
To learn more about pip
, visit our Python pip tutorial.