CSV File
CSV is the most popular file format for working with tabular data. It has a .csv
file extension, and when we load it to a text file (other than spreadsheets or tables), you will see each element will be separated by a single character (generally commas). For example,
Name, Age, Country
Maria, 21, Italy
Sudip, 26, Nepal
Sanjay, 19, India
Python CSV
Python provides a built-in module named csv that makes working with csv files a lot easier, and to use it, we must first import it.
import csv
After importing, we can perform file operations like Reading and Writing on the csv file.
1. Read CSV File
The csv
module provides a reader()
function to perform the read operation.
Suppose we have a csv file like this
Name, Age, Country
Maria, 21, Italy
Sudip, 26, Nepal
Sanjay, 19, India
Now, let's use the reader()
function to read this file.
import csv
with open('info.csv', 'r') as file:
csv_reader = csv.reader(file)
# read each row of the csv file
for row in csv_reader:
print(row)
Output
['Name', ' Age', ' Country'] ['Maria', ' 21', ' Italy'] ['Sudip', ' 26', ' Nepal'] ['Sanjay', ' 19', ' India']
In the above example, we have imported the csv
module in our program. We then open the info.csv file using open()
. This is similar to opening any file in Python. To learn more, visit Python files.
Here, we have used the csv.reader()
function to read the csv
file. This function returns an iterable object which we then accessed using a for
loop.
The above code works fine if each row of the csv file is separated by commas, which might not be the case all the time. Sometimes, in text editors, csv files are separated by the -
symbol or a tab.
In such cases, we have to provide the optional delimiter
argument to the reader()
function. For example, if we have a csv file where data is separated by tabs.
Name-Age-Country
Maria-21-Italy
Sudip-26-Nepal
Sanjay-19-India
Let's first see what happens with the older code
import csv
with open('info.csv', 'r') as file:
csv_reader = csv.reader(file)
# read each row of the csv file
for row in csv_reader:
print(row)
Output
['Name-Age-Country'] ['Maria-21-Italy'] ['Sudip-26-Nepal'] ['Sanjay-19-India']
Here, the code runs fine, but if you look into the output carefully, you can see the individual columns of the csv files are treated as a single element, 'Name-Age-Country'
, 'Maria-21-Italy'
, etc.
Now, let's use the delimiter parameter.
import csv
with open('info.csv', 'r') as file:
csv_reader = csv.reader(file, delimiter = '-')
# read each row of the csv file
for row in csv_reader:
print(row)
Output
['Name', 'Age', 'Country'] ['Maria', '21', 'Italy'] ['Sudip', '26', 'Nepal'] ['Sanjay', '19', 'India']
This time we get the desired output.
2. Write CSV FIle
Similar to the reader()
function, the csv module also provides the writer()
function to write data to a file.
Let's see an example,
import csv
with open('info.csv', 'w') as file:
csv_writer = csv.writer(file)
csv_writer.writerow(['Name', 'Age', 'Country'])
csv_writer.writerow(['Steve', 32, 'Australis'])
csv_writer.writerow(['Messi', 34, 'Argentina'])
csv_writer.writerow(['Ronaldo', 37, 'Portugal'])
Here, we have used the writer()
function to get a writer object, which we then used to call the writerow()
function. The writerow()
function writes the provided data to the csv file.
After running the above code, our info.csv file will look something like this.
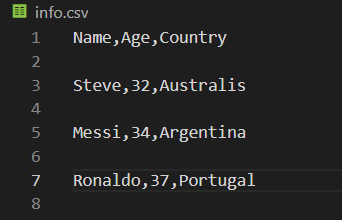
You can see there is one line after each row of data, which doesn't look good. Let's change it.
import csv
with open('info.csv', 'w', newline='') as file:
csv_writer = csv.writer(file)
csv_writer.writerow(['Name', 'Age', 'Country'])
csv_writer.writerow(['Steve', 32, 'Australis'])
csv_writer.writerow(['Messi', 34, 'Argentina'])
csv_writer.writerow(['Ronaldo', 37, 'Portugal'])
If you look carefully, you can see we have now added the newline=''
parameter to the open()
function. By default, it has \n
value, so we have seen the previous output.
Now when we run this code, our file will look like this:
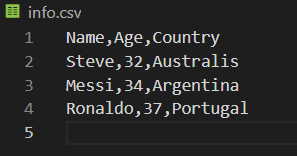
Write data with a delimiter
Instead of ,
as the delimiter, we can also provide a custom single character delimiter. For example,
import csv
with open('info.csv', 'w', newline='') as file:
csv_writer = csv.writer(file, delimiter = '-')
csv_writer.writerow(['Name', 'Age', 'Country'])
csv_writer.writerow(['Steve', 32, 'Australis'])
csv_writer.writerow(['Messi', 34, 'Argentina'])
csv_writer.writerow(['Ronaldo', 37, 'Portugal'])
info.csv file
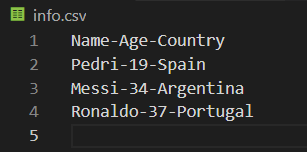
Note: When we perform the write operation on the csv file, the initial content of that file will be replaced by the new content. For example, before, our file looked like this.

And when we run the code with a delimiter, this content is replaced by
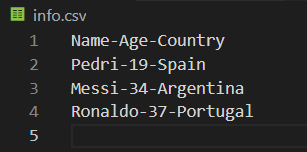
Writing List Content to CSV File
Instead of writing individual data, we can create a nested list and then write data from the list to our csv file. For example,
import csv
# list of data to be stored in the csv file
csv_data = [['Name', 'Age', "Country"], ['Pedri', 19, 'Spain'],
['Messi', 34, 'Argentina'], ['Ronaldo', 37, 'Portugal']]
with open('info.csv', 'w', newline='') as file:
csv_writer = csv.writer(file, delimiter = '-')
csv_writer.writerows(csv_data)
This time, we have used the writerows()
function because we are writing multiple rows at the same time. If we run the code, our info.csv file will look like this.
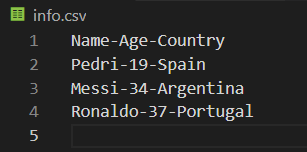
Python CSV with Dictionary
So far, we have been reading and writing csv files using Python List, now, let's use a dictionary to perform the read and write operations on csv.
1. Read CSV using Dictionary
We use the DictReader() function to read the csv file as a dictionary. For example,
info.csv
Name, Age, Country
Pedri, 19, Spain
Messi, 34, Argentina
Ronaldo, 37, Portugal
main.py
import csv
with open('info.csv', 'r') as file:
csv_reader = csv.DictReader(file)
for row in csv_reader:
print(row)
Output
{'Name': 'Pedri', ' Age': ' 19', ' Country': ' Spain'} {'Name': 'Messi', ' Age': ' 34', ' Country': ' Argentina'} {'Name': 'Ronaldo', ' Age': ' 37', ' Country': ' Portugal'}
As you can see we now get the result as a dictionary as the key/value pairs where data of the first row acts as keys and all other data acts as values.
2. Write CSV using Dictionary
Similarly, we can use the DictWriter()
to write data from a dictionary to a csv file. For example,
main.py
import csv
# create a list with dictionary nested inside it
csv_data = [ {'Name': 'Pedri', 'Age': 19, 'Country': 'Spain'}, {'Name': 'Messi', 'Age': 34, 'Country': 'Argentina'}, {'Name': 'Ronaldo', 'Age': 37, 'Country': 'Portugal'}
]
field_names = ['Name', 'Age', 'Country']
with open('info.csv', 'w', newline = '') as file:
csv_writer = csv.DictWriter(file, fieldnames = field_names)
csv_writer.writeheader()
csv_writer.writerows(csv_data)
info.csv
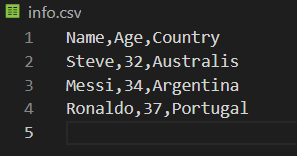
When we compare it to writing as a list, we have added
field_names
to specify the name of each field in the csv filewriteheader()
to write the header of the csv file
Read CSV using Panda
Besides csv
, Python also provides the panda module, which we can use to perform various operations on csv files. For example,
info.csv
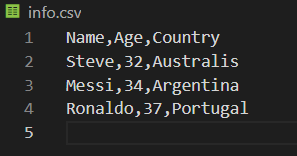
main.py
import pandas
print(pandas.read_csv('info.csv'))
Output
Name Age Country 0 Pedri 19 Spain 1 Messi 34 Argentina 2 Ronaldo 37 Portugal
Here, the read_csv()
function reads the content of the info.csv file. Similarly, we can also read csv content using pandas, which we will discuss in upcoming tutorials.