There are different ways to pass arguments to a Python function. They are as follows:
- Positional Arguments
- Default Arguments
- Keyword Arguments
Python Positional Arguments
The positional arguments are the most basic type of arguments passed to a function.
When you call a function and provide values for its parameters, those values are assigned to the parameters based on their position or order in the function's parameter list. For example,
def add_numbers(n1, n2):
result = n1 + n2
return result
result = add_numbers(5.4, 6.7)
print(result) # 12.100000000000001
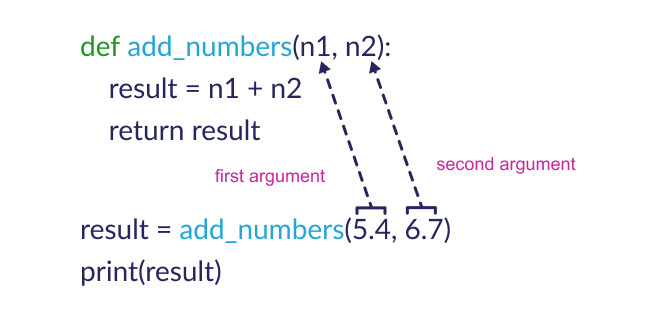
We have called the add_numbers()
function with two arguments.
- 5.4 - assigned to the first parameter n1
- 6.7 - assigned to the second parameter n2
These arguments are passed based on their position. Hence, are called positional arguments.
If we remove the second argument, it will lead to an error message:
def add_numbers(n1, n2):
result = n1 + n2
return result
result = add_numbers(5.4)
print(result)
The code throws the following error:
Traceback (most recent call last): File "<string>", line 5, in <module> TypeError: add_numbers() missing 1 required positional argument: 'n2'
This error message tells us that we must pass a second argument for n2 during the function call because our add_numbers()
function takes 2 arguments.
Python Default Arguments
Function arguments can have default values in Python. The default arguments are parameters in a function definition that have a predefined value assigned to them.
These default values are used when an argument for that parameter is not provided during the function call.
We can provide a default value to an argument by using the assignment operator =
. For example,
def add_numbers(n1 = 100, n2 = 1000):
result = n1 + n2
return result
result = add_numbers(5.4)
print(result) #1005.4
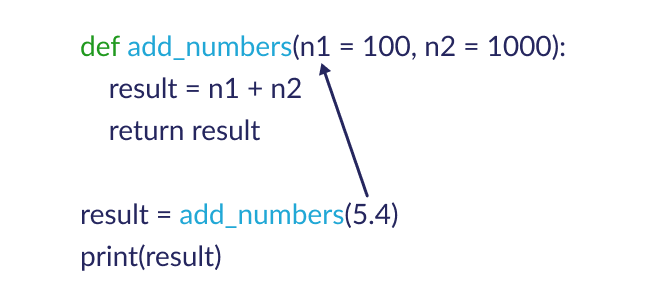
Here, n1 takes the value passed in the function call. Since there is no second argument in the function call, n2 takes the default value of 1000.
We can also run the function without any arguments:
def add_numbers(n1 = 100, n2 = 1000):
sum = n1 + n2
return sum
result = add_numbers()
print(result)
Output
1100
Keyword Arguments
In Python, keyword arguments are a way to pass arguments to a function using the parameter names explicitly.
Instead of relying on the order of the arguments, keyword arguments allow you to specify the argument along with its corresponding parameter name.
def greet(name, message):
print('Hello', name)
print(message)
# call the greet() function with positional arguments
greet('Jack', 'What is going on?')
# call the greet() function with keyword arguments
greet(message='Howdy?', name='Jill')
Output
Hello Jack What is going on? Hello Jill Howdy?
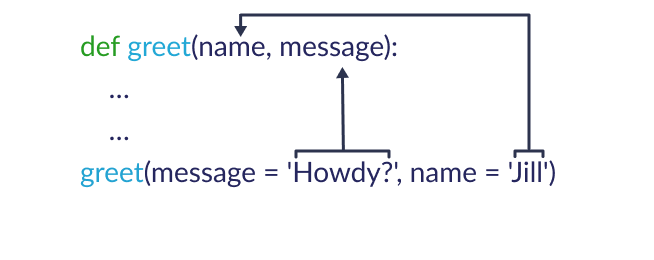
During the second function call greet(message='Howdy?', name='Jill')
, we passed arguments by their parameter name.
The string 'Howdy?'
is associated with the message
parameter, and the string 'Jill'
is associated with the name
parameter. When we call functions in this way, the order (position) of the arguments can be changed.