In Python, the match...case
statement allows us to execute a block of code among many alternatives.
The match...case
evaluates a given expression and based on the evaluated value, it executes the certain associated statement(s).
It was first introduced in Python 3.10 which is similar to the switch...case
statement in other programming languages.
Python match...case Statement
The syntax of the match...case
statement in Python is:
match expression:
case value1:
# statements
case value2:
# statements
...
...
case other:
# statements
Here, if the value of expression
matches with value1
, statements inside case value1
are executed.
And if the value of expression
matches with value2
, statements inside case value2
are executed.
However, if there is no match, statements of case other
are executed.
Python match...case Flowchart
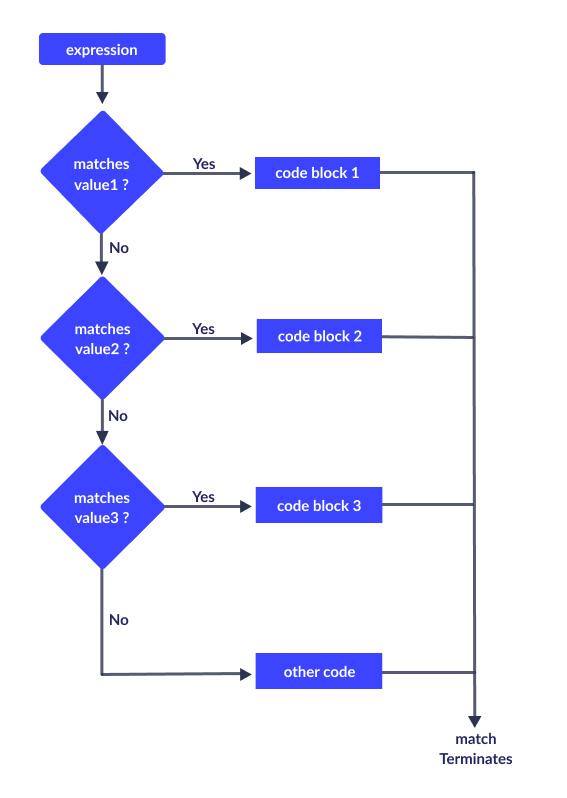
Example 1: Python match...case Statement
number = 48
match number:
case 29:
print('Small')
case 42:
print('Medium')
case 44:
print('Large')
case 48:
print('Extra Large')
case other:
print('Unknown')
# Output: Extra Large
Here, since the value matches with 48, the code inside case 48:
is executed.
Example 2: Python match…case With Tuple
In Python, we can also use tuples in match...case
statements. For example,
plot1 = (0, 4)
match plot1:
case (4,0):
print('on x-axis')
case (0,4):
print('on y-axis')
case (0,0):
print('center')
# Output: on y-axis
In the above example, we have created a tuple named plot1 with values: 0 and 4.
Here, instead of a single value, each case
statement also includes tuples.
Now, the value of plot1
is compared with each case
statement. Since the value matches with case (0,4)
, the statement inside it is executed.
To learn more about tuples, visit Python Tuple.
Using or(|) Statements in Cases
Python also lets us use or
statements in the cases. For example,
number = 2
match number:
case 2 | 3:
print('Small')
case 4 | 5 | 6:
print('Medium')
case 9 | 10:
print('Large')
# Output: Small
Here, in each case there is the |
(or) statement. It means that if the value of number matches with either of the values in a certain case, the code inside that case is executed.
other case in Python match...case
The match...case
statement also includes an optional other
case. It is executed when the expression doesn't match any of the cases. For example,
number = 20
match number:
case 29:
print('Small')
case 42:
print('Medium')
case 44:
print('Large')
case 48:
print('Extra Large')
case other:
print('Unknown')
# Output: Unknown
Here, the value of number doesn't match with any of the cases. So the statement inside case other:
is executed.
Note: We can use _
instead of other
as a default case as:
case _:
print(' Unknown')'
Using Python if statement in Cases
In Python, we can also use the if statement in case
. For example,
score = 84
match score:
case score if score >= 90:
print('A')
case score if score >= 80:
print('B')
case score if score >= 70:
print('C')
case score if score >= 60:
print('D')
case _:
print('F')
# Output: B
Here, we have used the if
statement in case
to match score and it will print out the first case it matches.
Recommended Reading: Python Booleans