In Python, there are three numeric types:
int
- Integersfloat
- Floating-point numberscomplex
- Complex numbers
Python int (integers)
An integer is a whole number (without decimal places). For example,
# 25 is an integer
age = 25
print(age) # 25
# -8 is an integer
scale = -8
print(scale) # -8
Python float
A floating-point number, float
, is a number with decimal places. For example,
# 3530.5 is a floating-point number
salary = 3530.5
print(salary) # 3530.5
# -25.0 is a floating-point number
number = -25.0
print(number) # -25.0
Note: -25 is an integer. However, -25.0 is a floating-point number because it has a decimal part.
Floating-point numbers are also used for exponential notation. For example,
value = 2e5
print(value) # 200000.0
Here, 2e5
is equal to 2 * 10^5
.
Python complex
A complex number is a number with real and imaginary parts. For example, 5 + 3.5j
is a complex number.
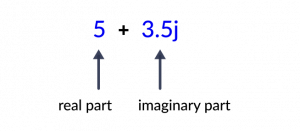
Python supports complex numbers by default. For example,
number1 = 5 + 3.5j
print(number1) # (5+3.5j)
Note: Complex numbers are rarely useful in everyday programming unless you work with mathematics.
Checking Type of Numbers
We can check the type of numbers and other values using the type()
function. For example,
# integer
x = 1
print(type(x)) # <class 'int'>
# floating-point number
y = 1.0
print(type(y)) # <class 'float'>
# complex number
z = 2j
print(type(z)) # <class 'complex'>
Type Conversion of Numbers
We can use
int()
to convert values to integersfloat()
to convert values to floating-point numberscomplex()
to convert values to complex numbers
a = 10 # int
b = 10.5 # float
# converting int to float
print(float(a)) # 10.0
# converting float to int
print(int(b)) # 10
# converting string to int
print(int('5')) # 5
# converting string to float
print(float('5.5')) # 5.5
To learn more, visit Python Type Conversion.