A function is a block of code that performs a specific task. They are a fundamental building block in Python programming and are essential for code reuse and organization.
Functions are used to break down large programs into smaller, modular components, making the code more organized, readable, and maintainable.
Declaring Function
def function_name(parameters):
# code
To create a function, we use the def
keyword followed by the function name, parenthesis ()
, and a colon :
. The body of the function is specified using indentation.
Let's see an example:
def greet():
print('Hello')
print('How do you do?')
Here, we have defined a function named greet()
.
When we run the program, we don't see any output. It is because just defining a function won't do anything. To bring the function into action, we need to call it.
Calling a Function
In the above program, we declared a function named greet()
. To use that function, we need to call it.
We call the function using the name of the function:
def greet():
print('Hello')
print('How do you do?')
# calling the greet() function
greet()
Output
Hello How do you do?
Here,
greet()
calls the greet()
function.
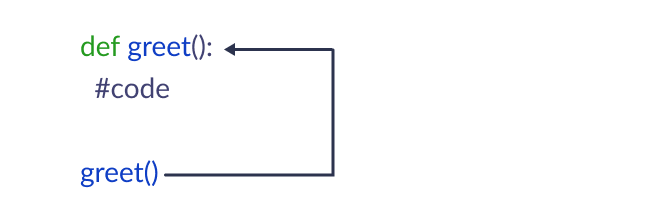
Multiple Function Call
One advantage of defining a function is that we can call it any number of times.
def greet():
print('Hello')
print('How do you do?')
# calling the greet() function 3 times
greet()
greet()
greet()
Output
Hello How do you do? Hello How do you do? Hello How do you do?
Note: We need to define the function before calling it.
Function Arguments
Suppose we want to make our greet()
function a bit more personal.
Instead of printing Hello, we want to print something like Hello Jack
or whatever the person's name is.
For this, we can use function arguments:
def greet(name):
print('Hello', name)
print('How do you do?')
greet('Jack')
Output
Hello Jack How do you do?
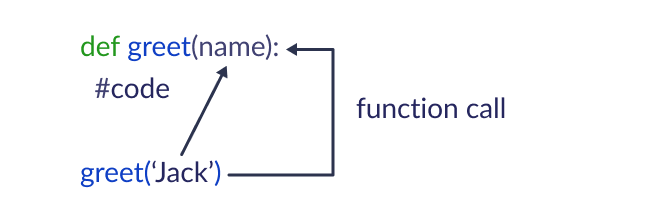
Function arguments are passed inside the parenthesis during the function call. It can then be accessed using the name parameter in the function definition.
Passing Multiple Arguments
If we need to pass multiple arguments to a function, we can separate them by commas.
Let's create a function to add two numbers.
def add_numbers(n1, n2):
result = n1 + n2
print("The sum is", result)
number1 = 5
number2 = 6
add_numbers(number1, number2)
Output
The sum is 11
We have passed number1 and number2 as arguments to the add_numbers()
function. These arguments are accepted as n1 and n2 once they are passed to the add_numbers()
function.
Return Value from Function
def add_numbers(n1, n2):
result = n1 + n2
print("The sum is", result)
add_numbers(5, 6)
The add_numbers()
function prints the sum of two numbers. However, it is better just to find the sum inside the function and print result somewhere else.
We can achieve that by using the return
statement.
def add_numbers(n1, n2):
result = n1 + n2
return result
result = add_numbers(5, 6)
print("The sum is", result)
Output
The sum is 11
Recommended Reading: Python pass statement