In Python, a tuple is similar to a list with one important difference. We cannot change elements of a tuple once it is assigned, whereas we can change items of a list.
Creating Tuples
A tuple is created by placing items inside ()
, separated by commas. For example,
# a tuple with 3 items
numbers = (1, 2, -5)
# tuple containing duplicate values
numbers = (1, 2, -5, 2)
# empty tuple
numbers = ()
In Python, a tuple may contain items of different types. For example,
# a tuple with 3 items of different types
random_tuple = (5, 2.5, 'Hello')
A tuple may also contain another tuple and other collection types as an element. For example,
# a tuple with two items: 5 and (4, 5, 6)
random_tuple = (5, (4, 5, 6])
Access Tuple Items
The items of a tuple are in order, and we can access individual items of a tuple using indexes. For example,
cars = ('BMW', 'Tesla', 'Ford')
# access the first item
print(cars[0]) # BMW
# access the third item
print(cars[2]) # Ford
In Python, indexing starts from 0. Meaning,
- the index of the first item is 0
- the index of the second item is 1
- and so on
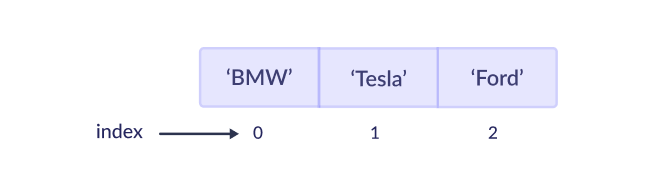
Hence, cars[0]
gives the first item of the cars
tuple, cars[1]
gives the second item, and so on.
Negative Indexing
Python tuples also support negative indexing.
- the index of the last item is -1
- the index of the second last item is -2
- and so on
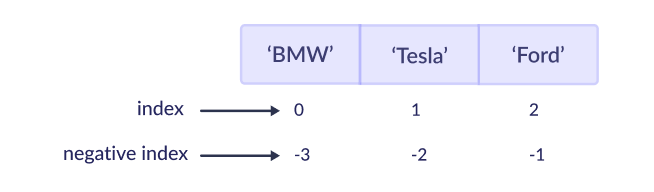
cars = ('BMW', 'Tesla', 'Ford')
# access the last item
print(cars[-1]) # Ford
# access the third last item
print(cars[-3]) # BMW
By the way, if the specified index does not exist in the tuple, Python throws the IndexError
exception.
cars = ('BMW', 'Tesla', 'Ford')
# trying to access the fourth item
# however, the tuple only has three items
print(cars[3])
Output
Traceback (most recent call last): File "<string>", line 5, in <module> IndexError: tuple index out of range
Slicing of a Tuple
It is also possible to access a section of items from the tuple, not just a single item (using the slicing notation). For example,
languages = ('Python', 'JavaScript', 'C++', 'Kotlin')
# first three items -> 0, 1, 2
print(languages[0: 3]) # ('Python', 'JavaScript', 'C++')
# second to last items -> 1, 2, 3
print(languages[1: 4]) # ('JavaScript', 'C++', 'Kotlin')
One thing to remember about tuple slicing is that the start index is inclusive whereas the end index is exclusive.
Hence,
languages[0: 3]
returns a tuple with items at index 0, 1, and 2 but not 3.languages[1: 4]
returns a tuple with items at index 1, 2, and 3 but not 4.
Slicing: Skip Start and End Index
If we use the empty start index, the slicing starts from the beginning of the tuple. Similarly, if we use the empty end index, the slicing ends at the last index.
languages = ('Python', 'JavaScript', 'C++', 'Kotlin')
# elements from index 0 to 1
print(languages[:2]) # ('Python', 'JavaScript')
# elements from index 2 to last index
print(languages[2:]) # ('C++', 'Kotlin')
Change Tuple Items
Python tuples are immutable. Meaning we cannot change items of a tuple once created.
If we try to change items of a tuple, we will get an error.
cars = ('BMW', 'Tesla', 'Ford')
# trying to change the second item
# Error: 'tuple' object does not support item assignment
cars[1] = 'Toyota'
print(cars)
Delete Tuple
We cannot remove items of a tuple because tuples are immutable. However, we can delete the tuple itself using the del
statement.
cars = ('BMW', 'Tesla', 'Ford', 'Toyota')
# deleting the cars tuple
del cars
print(cars) # Error: name 'cars' is not defined
Python Tuple Length
We can find the length of a tuple using the len()
built-in function. For example,
cars = ('BMW', 'Tesla', 'Ford', 'Toyota')
print(len(cars)) # 4
Python Tuple Methods
Since tuples are immutable, there are only two tuple methods.
Method | Description |
---|---|
index() |
returns the index of the first matched item |
count() |
returns the count of the specified item in the tuple |
Iterating through a Tuple
It's really easy to iterate through a tuple using the for loop.
cars = ('BMW', 'Tesla', 'Ford', 'Toyota')
# iterating through the tuple
for car in cars:
print(car)
Output
BMW Tesla Ford Toyota
Check if an Item Exists in the Tuple
We can use the in
keyword to check if an item exists in the tuple or not.
cars = ('BMW', 'Tesla', 'Ford', 'Toyota')
print('Kia' in cars) # False
print('Kia' not in cars) # True
print('BMW' in cars) # True
Use of Tuples
If we are sure that the items of a collection will not be changed, it's better to use tuples instead of lists.
It's because if we accidentally modify the items of a tuple, we will get an error. This helps to reduce bugs in our application.
Python Tuple Summary
In Python, a tuple is
- collection - can contain multiple items
- ordered - items of a tuple are in order
- unchangeable - items of a tuple can be changed
Recommended Reading: Python list